Next Steps¶
Using Your New Server¶
Once you have a server running, you can access it via its API or using the Python SDK. You can also use it to build Workflows using the Roboflow Platform UI.
Install the SDK¶
pip install inference-sdk
Run a workflow¶
This code runs an example model comparison Workflow on an Inference Server running on your local machine:
from inference_sdk import InferenceHTTPClient
client = InferenceHTTPClient(
api_url="http://localhost:9001", # use local inference server
# api_key="<YOUR API KEY>" # optional to access your private data and models
)
result = client.run_workflow(
workspace_name="roboflow-docs",
workflow_id="model-comparison",
images={
"image": "https://media.roboflow.com/workflows/examples/bleachers.jpg"
},
parameters={
"model1": "yolov8n-640",
"model2": "yolov11n-640"
}
)
print(result)
From a JavaScript app, hit your new server with an HTTP request.
const response = await fetch('http://localhost:9001/infer/workflows/roboflow-docs/model-comparison', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
// api_key: "<YOUR API KEY>" // optional to access your private data and models
inputs: {
"image": {
"type": "url",
"value": "https://media.roboflow.com/workflows/examples/bleachers.jpg"
},
"model1": "yolov8n-640",
"model2": "yolov11n-640"
}
})
});
const result = await response.json();
console.log(result);
Warning
Be careful not to expose your API Key to external users (in other words: don't use this snippet in a public-facing front-end app).
Using the server's API you can access it from any other client application. From the command line using cURL:
curl -X POST "http://localhost:9001/infer/workflows/roboflow-docs/model-comparison" \
-H "Content-Type: application/json" \
-d '{
"api_key": "<YOUR API KEY -- REMOVE THIS LINE IF NOT FILLING>",
"inputs": {
"image": {
"type": "url",
"value": "https://media.roboflow.com/workflows/examples/bleachers.jpg"
},
"model1": "yolov8n-640",
"model2": "yolov11n-640"
}
}'
Tip
ChatGPT is really good at converting snippets like this into other languages. If you need help, try pasting it in and asking it to translate it to your language of choice.
The easiest way to build and test your Workflows is by using the Roboflow Platform's visual debugger but you can also build with our Python SDK or start by forking an Example project.
Once you've built a Workflow you're happy with, you can set it up to run on a video stream.
Tutorials¶
You may also want to check out one of our Video Tutorials showing the end to end creation of a computer vision project with Inference:
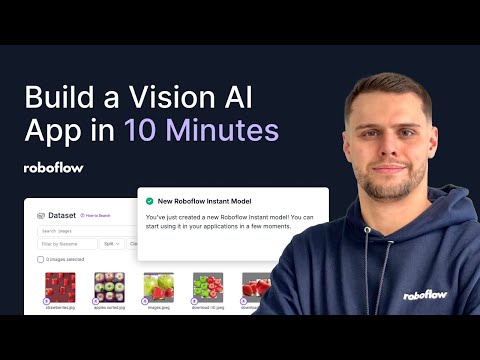
Make a computer vision app that identifies different pieces of hardware, calculates the total cost, and records the results to a database.
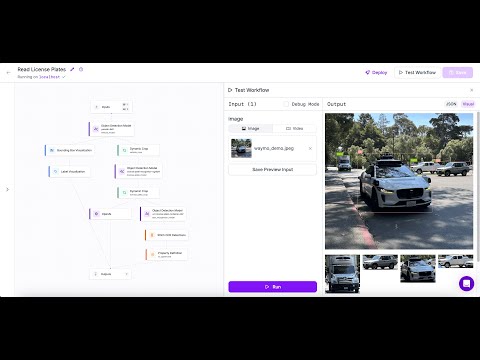
Learn how to build and deploy Workflows for common use-cases like detecting vehicles, filtering detections, visualizing results, and calculating dwell time on a live video stream.
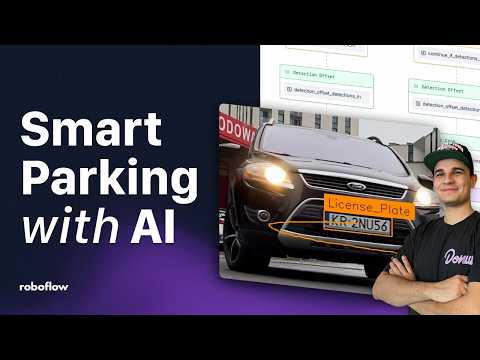
Build a smart parking lot management system using Roboflow Workflows! This tutorial covers license plate detection with YOLOv8, object tracking with ByteTrack, and real-time notifications with a Telegram bot.