background_color
BackgroundColorAnnotator
¶
Bases: BaseAnnotator
A class for drawing background colors outside of detected box or mask regions.
Warning
This annotator uses sv.Detections.mask
.
Source code in inference/core/workflows/core_steps/visualizations/common/annotators/background_color.py
7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
|
__init__(color=Color.BLACK, opacity=0.5, force_box=False)
¶
Parameters:
Name | Type | Description | Default |
---|---|---|---|
color |
Color
|
The color to use for annotating detections. |
BLACK
|
opacity |
float
|
Opacity of the overlay mask. Must be between |
0.5
|
Source code in inference/core/workflows/core_steps/visualizations/common/annotators/background_color.py
14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
annotate(scene, detections)
¶
Annotates the given scene with masks based on the provided detections.
Args:
scene (ImageType): The image where masks will be drawn.
ImageType
is a flexible type, accepting either numpy.ndarray
or PIL.Image.Image
.
detections (Detections): Object detections to annotate.
Returns:
The annotated image, matching the type of scene
(numpy.ndarray
or PIL.Image.Image
)
Example:
import supervision as sv
image = ...
detections = sv.Detections(...)
background_color_annotator = sv.BackgroundColorAnnotator()
annotated_frame = background_color_annotator.annotate(
scene=image.copy(),
detections=detections
)
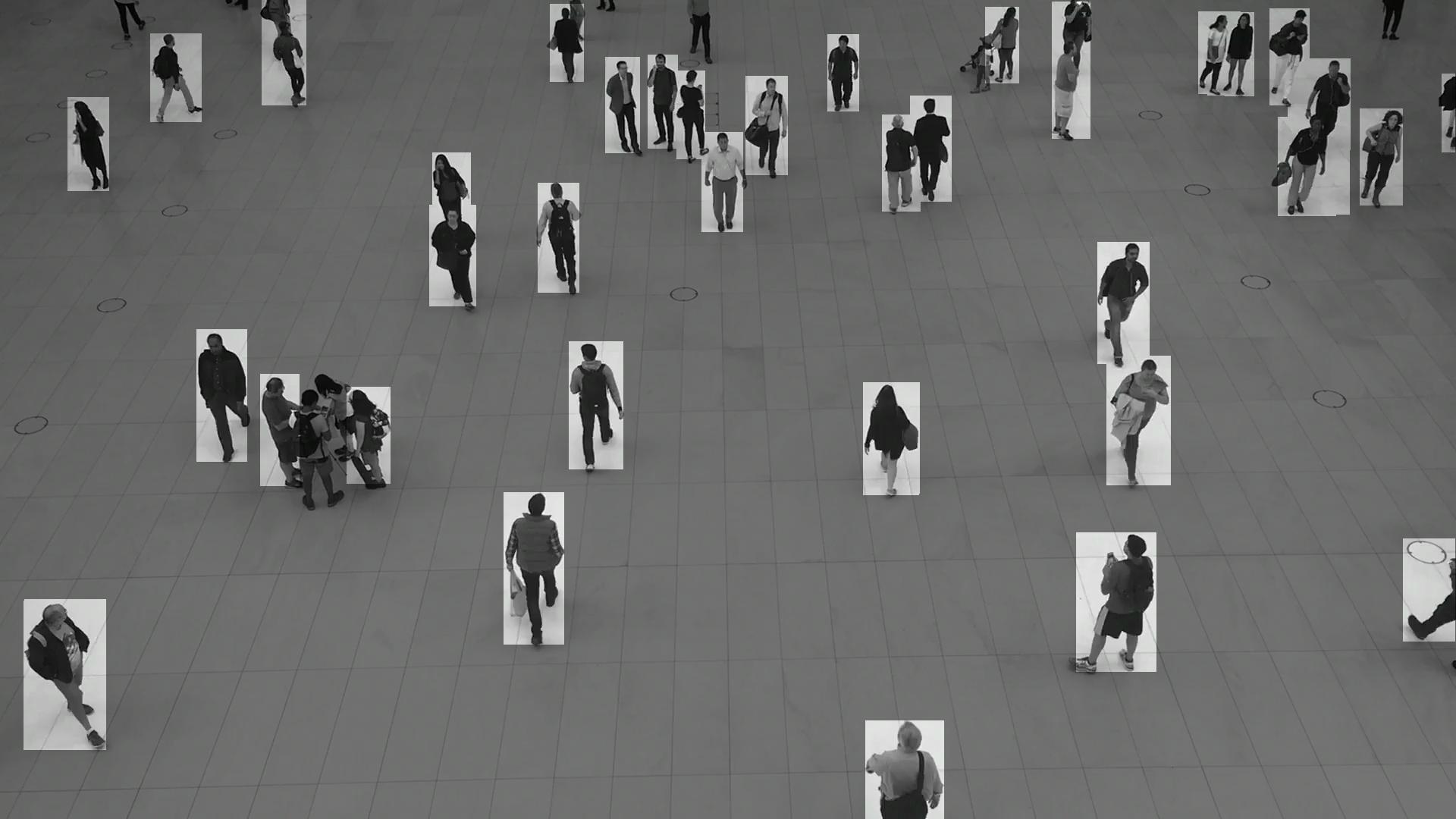
Source code in inference/core/workflows/core_steps/visualizations/common/annotators/background_color.py
29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
|